Swift is a new high-performance compiled language designed by Apple. I’ve had some experience using it for an IOS development project, but the language is open source and is already available for Linux.
Some of you are probably able to appreciate the irony of me writing a blog post about Apple’s new programming language Swift, but here we are. :) I am, grudgingly, really impressed by Apple’s good work.
In this post I’m going to chat about closure expression syntax, in the process showing you IBM’s Swift Sandbox, an online tool for experimenting with Swift.
Let’s start with a simple example (slightly modified from Apple’s documentation on Swift closures) for sorting a list of strings in reverse alphabetical order:
// names will be constaint, so we use let and not var let names = ["Chris", "Alex", "Ewa", "Barry", "Daniella"]// function taking two parameters and returning boolean func backwards(s1: String, _ s2: String) -> Bool { return s1 > s2 }
let reversed = names.sort(backwards)
print(“Reversed alpha:", reversed)
That’s a complete compilable program in Swift, which you can run directly in your browser by clicking here.
We use let
to define a list of strings. Swift is strongly-typed, but in this case it simply infers the type of the names
variable from the value that’s assigned to it. MAGIC!
If we were planning to change the value of the names
list at some point, we would have had to use var
(mutable) instead of let
(immutable).
We then define a function called backwards
that takes two strings, and returns the true value if the first is larger than the second. In the final let statement, we call the sort()
method on the names list, but we pass it the backwards()
function, which it will use to compare elements during sorting. Because we have flipped the s1
and s2
variables, we get our list back in reverse alphabetical order.
sort()
can be seen as a higher order function that takes the backwards()
function as one of its inputs, and uses it to do its work.
Since we’re only using the backwards()
function once, it would have been pretty convenient if there were some way to define it more compactly and in-place.
This is exactly what Swift’s closure expression syntax
is for. Here’s the above code, with the backwards()
function written as a closure expression:
let names = ["Chris", "Alex", "Ewa", "Barry", "Daniella"]// closure expression syntax for inline anonymous function: // { (args) -> returnType in statements } var reversed = names.sort({ (s1: String, s2: String) -> Bool in return s1 > s2})
print(“Reversed:", reversed)
(You can run this Swift code in your browser by clicking here.)
Instead of having to define and name a separate function, we can specify a function in the exact spot where it’s needed, using closure expression syntax i.e.: { (parameters) -> returnType in statements }
.
For you Python-heads out there (MY PEOPLE!), the above code can be written in Python as follows:
names = ["Chris", "Alex", "Ewa", "Barry", "Daniella"]reversed = sorted( names, cmp=lambda s1, s2: 0 if s1 == s2 else (-1 if s1 > s2 else 1))
print(reversed)
In Python, lambda
is used to specify anonymous function objects (our closure expression syntax, in other words). The compare function is defined differently, which is why it’s somewhat more complicated, but the principle is the same.
Whilst typing this blog, I downloaded Swift 2.2 from the open source download site and installed it on my Ubuntu 14.04 laptop (the internet is so slow over here, we have to multi-task to stay sane). After compiling both the examples using swiftc whatever.swift
, the resulting binaries are about 21 Kbytes each (they are dynamically linked to a number of system libraries, and the 5M libswiftCore.so
).
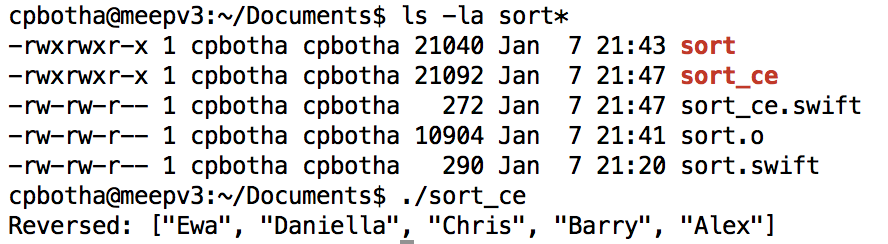
The Swift language is open source, is already available for Linux, has a number of really compelling modern language features of which closure expressions are just one example (other interesting examples include optional types and protocol extensions), is statically typed with good type inference, and has a good compiler. For these reasons, it should be strongly considered for a permanent spot in your compiled language toolbox!
P.S. Yes, I do know that C++ has lambda
and auto
. I like them too!